Introduction
JupyterLab on ArenaView enables application designers to quickly test and validate all camera features and speed up their product qualification workflow without having to setup a custom development environment. JupyterLab provides a built-in and preconfigured interactive development environment that can be used for testing and documenting all SDK functions and camera performances. It is installed with Arena SDK using the Developer installation option. The following instructions explains how to launch and use JupyterLab within ArenaView.
Requirements
Arena SDK:
- ArenaView v1.0.33.0 or newer
Initial Setup
Please make sure you have read and completed the initial setup section found in the Arena SDK documentation. In particular, make sure you have turned on jumbo frames and set your receive buffer size. Atlas10 users that plan to use TCP should read and complete our setup guide for using TCP with Atlas10 cameras.
Enabling JupyterLab Server
Step 1: Open the Options Window
Open the Options Windows by clicking View -> Toolbar -> Options.

Step 2: Select the JupyterLab Options tab
In the Options Window, click the hamburger button and choose the JupyterLab tab.


Step 3: Turn on the JupyterLab server
In the Jupyter Lab options tab, turn on the Jupyer Lab server. If this is your first time installing Jupyter Lab, ArenaView will download and install the required dependencies.
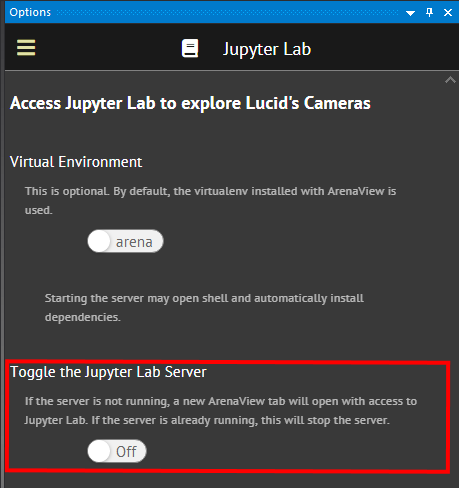
Step 4: Enter the JupyerLab server token or create a server password
If this is your first time installing JupyterLab, you will also be asked for a token to access the JuptyerLab server. This token is presented after ArenaView installs the JupyterLab dependencies. Enter the token into the “Password or token” field and press Log in. You can also assign a server password instead of using a token.

Opening Jupyter Notebooks
After starting the JupyterLab server, you will be presented with the Jupyer launch page. From this page, you can start your own Jupyter notebook or browse the preinstalled examples that come with the Arena SDK installation.
By default, Jupyter notebooks are found at C:\ProgramData\LUCID Vision Labs\ArenaView\ArenaJp.

Using Jupyter Notebooks
The Jupyter Lab environment will let you write Arena Python code to control camera features and quickly integrate image analysis and processing.
Jupyter Code Cells
A Jupyter code cell contains Python code that is executed by the Jupyter kernel. When the cell type is set to Code:
- Press the + button to insert a new code cell. This will place a new cell under the currently selected cell.
- Press the ▶ button to execute a code cell. The code cell will display an asterisk (*) beside the cell while it is being executed, and it will show a number upon completion.




Jupyter Markdown Cells
Formatted text can be entered in a Jupyter notebook for documentation.
Formatted text can be entered in a Jupyter notebook for displaying visually rich documentation. When the cell type is set to Markdown:
- Press the + button to insert a new markdown cell. This will place a new cell under the currently selected cell.
- Press the ▶ button to execute a markdown cell. The markdown cell will be formatted for display.


SDK Documentation
The Arena SDK Python documentation can be found at the ArenaView Start Page (View -> Start Page):
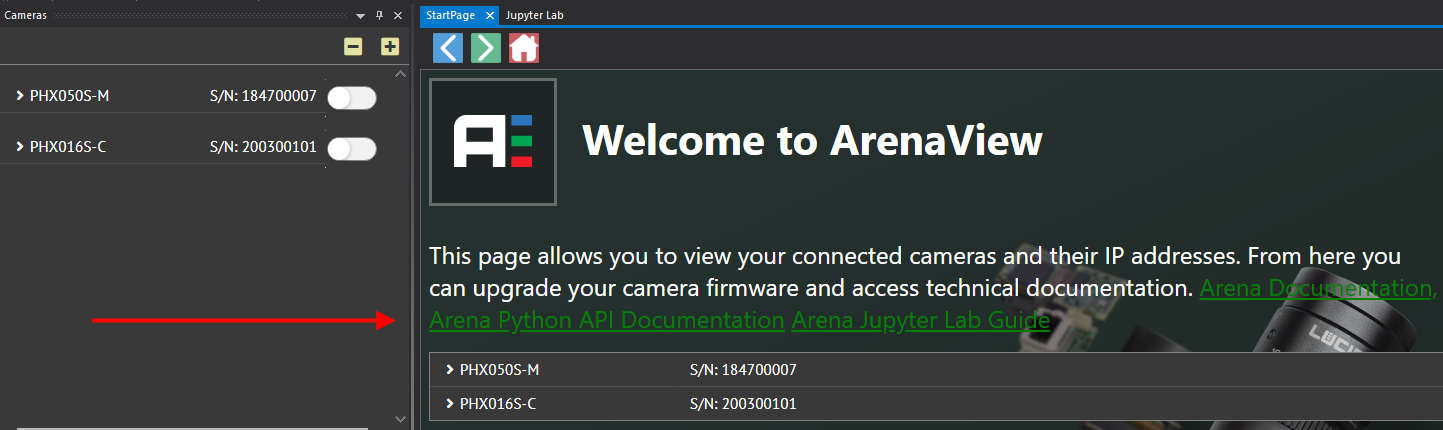
Within a Jupyter notebook, it is also possible to display a function’s documentation by adding ?? at the end of a function:

Sample Code Snippets
Create Device By Serial Number
The following code snippet shows how to connect to a camera by its serial number.
def create_device_from_serial_number(serial_number): camera_found = False device_infos = None selected_index = None device_infos = system.device_infos for i in range(len(device_infos)): if serial_number == device_infos[i]['serial']: selected_index = i camera_found = True break if camera_found == True: selected_model = device_infos[selected_index]['model'] print(f"Create device: {selected_model}...") device = system.create_device(device_infos=device_infos[selected_index])[0] else: raise Exception(f"Serial number {serial_number} cannot be found") return device
Connect to the camera by calling:
device = select_device_from_serial_number("192900063")
Convert Image into OpenCV
The following code snippet shows how to convert an image into OpenCV:
!pip install opencv-python [...] import cv2 [...] image_buffer = device.get_buffer() nparray = np.ctypeslib.as_array(image_buffer.pdata,shape=(image_buffer.height, image_buffer.width, int(image_buffer.bits_per_pixel / 8))).reshape(image_buffer.height, image_buffer.width, int(image_buffer.bits_per_pixel / 8))
Convert a Jupyter Notebook to an Executable Program
ArenaView allows users to generate an executable program out of their Jupyter notebook.
The following steps show converting the included Jupyter notebook, py_acquisition.ipynb, into an executable file.
Step 1: Run the following command in your Jupyter notebook:
!jupyter nbconvert --to script py_acquisition.ipynb
This command will create a py file in the current directory:
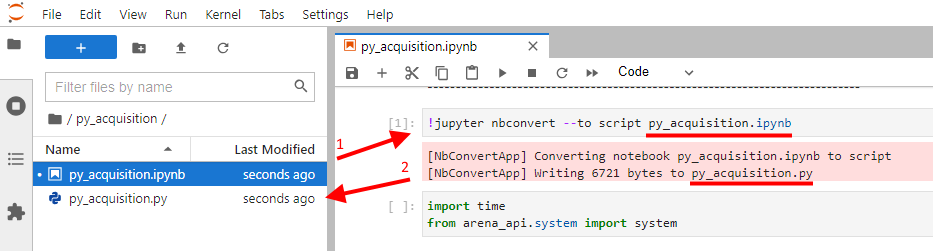
Step 2: Select the py file to convert to an exe file in the JupterLab Options
Step 3: Convert the py file to an exe file in the JupterLab Options

When you click the Convert button, you will be asked if you want to edit the pytoexe.bat file.

Editing the pytoexe.bat file allows you to add additional paths and binaries to the pyinstaller command to ensure your exe file works correctly.
Editing this file is necessary if:
- You are using a custom virtual environment
- You have downloaded additional libraries to the default environment, and are using the new libraries in your program
When the conversion is complete, you will be prompted to press a key to continue. This will open a window to the location of your new exe file.


The arena_api wheel used by the ArenaJp virtual environment can be found at C:\ProgramData\LUCID Vision Labs\ArenaView\ArenaPy.
The wheel file can be installed with a command similar to the following:
pip install arena_api-<>.<>.<>-py3-none-any.whl
Using a Custom Virtual Environment
By default, ArenaView uses a preinstalled Miniconda virtual environment for the JupyterLab server. The preinstalled virtual environment is found at: C:\ProgramData\LUCID Vision Labs\ArenaView\ArenaPy.
It is possible to define the virtual environment used by ArenaView in the JupyerLab Options tab. Set the virtual environment slider to arena to use the preinstalled virtual environment:

Set the virtual environment slider to venv and press the Browse button to select the to your custom virtual environment:

Custom virtual environments must install the JupyterLab requirements in the jupyter_requirements_win.txt file found at C:\ProgramData\LUCID Vision Labs\ArenaView\ArenaPy:
pip install -r jupyter_requirements_win.txt